
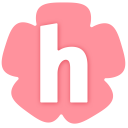
Posts tagged with :spring-of-making:



























systemd
and dbus
, which are not even used atm
















systemd
enabled Fedora 42 CoreOS container image with RPi Web Shell built-in! Not gonna bake and export the image because last time it crashed my entire Pi (it's a Pi 5 with 8g of RAM, but apparently the uSD cannot endure it...). However, i will put the completed Dockerfile
on the repo! THATS WHY I NEED SSD ASSESORIES!!! 


